GETTING STARTED WITH CSS - STYLING HTML ELEMENTS
GETTING STARTED WITH CSS - STYLING HTML ELEMENTS
GETTING STARTED WITH CSS - STYLING HTML ELEMENTS
CSS (Cascading Style Sheets) is used to style and lay out web pages for example. They can be used to alter the font, color, size, and spacing of content, you can add animations and other decorative features. This article will guide you towards CSS mastery with the basics of how it works, what the syntax looks like, and how you can start using it to add styling to HTML elements on a web page.
PREREQUISITES
Prerequites are conditions that must be met for an event to occur, before starting this article, you should have a very good understanding of the following:
- Familiarity with computers and using IDE to code.
- Basic knowledge of html and its attributes, knowledge of semantics will also be a very good advantage for you when styling.
TOPICS
In this article, we will look at the following css concepts. Bear in mind that you are expected to style some html elements at the end of this article.
- Looking at HTML.
- Adding CSS to our html.
- Styling HTML elements.
- Adding default behavior.
- Adding classes to elements..
LOOKING AT HTML
Our starting point is an HTML document. You can copy the code from below if you want to work on your own computer. Save the code below as index.html in a folder on your machine.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>Getting started with CSS</title>
</head>
<body>
<h1>I am a level one heading</h1>
<p>
This is a paragraph of text. In the text is a
<span>span element</span> and also a
<a href="https://example.com">link</a>.
</p>
<p>
This is the second paragraph. It contains an <em>emphasized</em> element.
</p>
<ul>
<li>Item <span>one</span></li>
<li>Item two</li>
<li>Item <em>three</em></li>
</ul>
</body>
</html>
You can remember from your html introductions, the syntax of html and all the tags required, let us now look at a way to add css to our html document.
ADDING CSS TO OUR HTML DOCUMENT
The first thing we need to do is to tell the HTML document that we have some CSS rules we want to use. There are different ways to apply CSS to an HTML document but for now, we will look at the most common way of doing so. Linking CSS from the head of your document.
Create a file in the same folder as your HTML document and save it as styles.css. The .css extension shows that this is a CSS file, it is very important and should not be omitted for any reason.
To link styles.css to index.html, add the following line somewhere inside the <head> of the HTML document:
<link rel="stylesheet" href="styles.css" />
This <link> tag tells the browser that we have a CSS stylesheet using the rel attribute, and the location of that stylesheet as the value of the href attribute. Recall that the href attributes points to link when using the <a> tag. You can test that the CSS works by adding a rule to styles.css. Got to your css file and add the following code :
h1 {
color: red;
}
Save your HTML and CSS files and reload the page in a web browser. The level one heading at the top of the document should now be red. If you read the CSS syntax, you will clearly see how the h1 element in the html was targeted and then styled. If the styling happens, you have successfully applied some CSS to an HTML document. If that doesn't happen, carefully check that you've typed everything correctly. It is very common for beginners to make mistakes with syntax errors when coding CSS.
STYLING HTML ELEMENTS
By making our heading red, we have already demonstrated that we can target and style an HTML element. We do this by targeting an element selector this is a selector that directly matches an HTML element name. To target all paragraphs in the document, you would use the selector p. To turn all paragraphs green, you would use:
p {
color: green;
}
You can target multiple selectors at the same time by separating the selectors with a comma. If you want all paragraphs and all list items to be green, your rule would look like this:
p,li {
color: green;
}
ADDING DEFAULT BEHAVIOR TO ELEMENTS
When we look at a simple html element we can see how the browser is making the HTML readable by adding some default styling. Headings are large and bold and our list has bullets. This happens because browsers have internal style sheets containing default styles for html elements, which they apply to all pages by default; without them all of the text would run together in a clump and we would have to style everything from scratch. Most modern browsers display HTML content by default in pretty much the same way.
However, most times you will want to customize your html elements differently from the browser styling format. This can be done by choosing the HTML element that you want to change and using a CSS rule to change the way it looks. A good example is <ul>, an unordered list. It has a list of bullets, If you don't want those bullets, you can remove them like so:
li {
list-style-type: none;
}
ADDING CLASSES TO ELEMENTS
For most of the article, we have styled elements based on their HTML element names. This works as long as you want all of the elements of that type in your document to look the same. Most of the time that isn't the case, you will need to find a way to select a particular element you want to style without changing the others. The most common way to do this is to add a class to your HTML element and target that class, this then makes the element unique for specific styling.
In your HTML document, add a class attribute to the second list item. Your list will now look like this:
<ul>
<li>Item one</li>
<li class="special">Item two</li>
<li>Item <em>three</em></li>
</ul>
In your CSS, you can target the class of special by creating a selector that starts with a full stop character. Add the following to your CSS file:
.special {
color: orange;
font-weight: bold;
}
You can see how the “.” syntax works, it is always placed in front of the class selector in css and then styled.
STYLING BASED ON LOCATION IN DOCUMENT
There are times when you will want something to look different based on where it is in the document. There are a number of selectors that can help you here, but for now we will look at just a couple. In our document, there are two <em> elements — one inside a paragraph and the other inside a list item. To select only an <em> that is nested inside an <li> element, you can use a selector called the descendant combinator, which takes the form of a space between two other selectors.
Add the following rule to your stylesheet:
li em {
color: rebeccapurple;
}
This selector will select any <em> element that is inside (a descendant of) an <li>. So in your example document, you should find that the <em> in the third list item is now purple, but the one inside the paragraph is unchanged.
STYLING THINGS BASED ON STATE
The final type of styling we shall take a look at in this tutorial is the ability to style things based on their state. A straightforward example of this is when styling links. When we style a link, we need to target the <a> (anchor) element. This has different states depending on whether it is unvisited, visited, being hovered over, focused via the keyboard, or in the process of being clicked (activated). You can use CSS to target these different states; the CSS below styles unvisited links pink and visited links green.
a:link {
color: pink;
}
a:visited {
color: green;
}
You can change the way the link looks when the user hovers over it, for example by removing the underline, which is achieved by the next rule:
a:hover {
text-decoration: none;
}
SUMMARY
In this article, we have taken a look at a number of ways in which you can style a document using CSS. We will be developing this knowledge as we move through the rest of the lessons. However, you now already know enough to style text, apply CSS based on different ways of targeting elements in the document. Remember to practise as you will get better with. Check out online resources for css tasks to improve your skills.
What's Your Reaction?
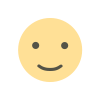
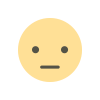
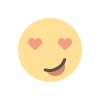
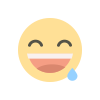
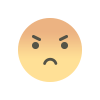
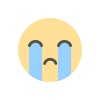
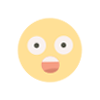